To convert HEX to RGB to HSL in VSCode, you can use the “Convert Color Format” extension. Colors can be represented in different formats depending on the context. For example, on the web in CSS, you can represent colors using RGB, HSL, HEX, and HSB. In this tutorial, we will learn how to convert HEX colors to RGB and HSL in Visual Studio Code. We will also explore some other useful color conversion formulas that can be useful for web development.
HEX stands for hexadecimal, and it is a way of representing colors using a six-digit code. The code consists of three pairs of characters, each representing the intensity of the red, green, and blue (RGB) channels, respectively. HEX codes are often used in web development to specify colors.
Table of Contents
RGB stands for Red Green Blue, and it is a color model that represents colors using a combination of red, green, and blue light. In this model, each color channel can have a value between 0 and 255, with 0 representing the absence of the color and 255 representing the maximum intensity of the color.
HSL stands for Hue Saturation Lightness, and it is another color model that represents colors using a combination of hue, saturation, and lightness. In this model, hue represents the color itself (e.g. red, green, blue, etc.), saturation represents the intensity of the color, and lightness represents the brightness of the color.
Here’s how:
Open VS Code and press Ctrl+Shift+X to open the Extensions pane.
In the search box, type “Convert Color Format” and press Enter.
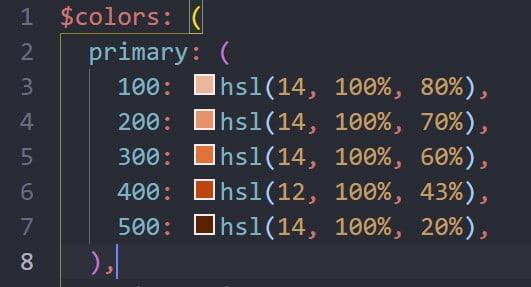
convert HEX to RGB to HSL in VSCode
Click the “Install” button to install the extension.
Once the extension is installed, open a file that contains a HEX color code. For example: #FF0000
Select the HEX color code, right-click, and choose “Convert Color Format” from the context menu.
In the “Convert Color Format” menu, select the format you want to convert to (e.g. RGB or HSL).
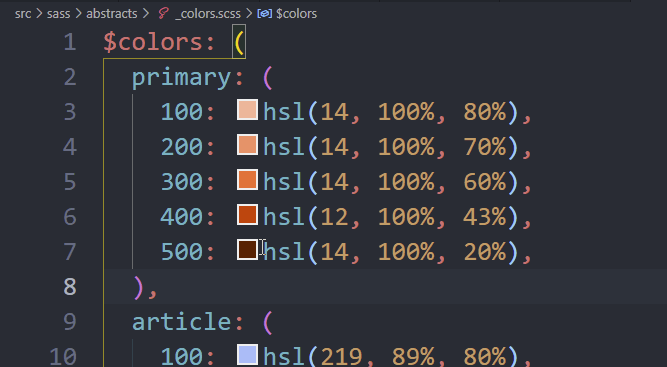
The extension will convert the selected HEX color code to the specified format.
Alternatively, you can also use online tools or code snippets to convert HEX to RGB or HSL. For example, you can use the following JavaScript code to convert a HEX color code to RGB:
function hexToRgb(hex) {
var result = /^#?([a-f\d]{2})([a-f\d]{2})([a-f\d]{2})$/i.exec(hex);
return result ? {
r: parseInt(result[1], 16),
g: parseInt(result[2], 16),
b: parseInt(result[3], 16)
} : null;
}
To use this function, pass in a HEX color code as a string (e.g. hexToRgb('#FF0000')
) and it will return an object with the RGB values (e.g. { r: 255, g: 0, b: 0 }
).
How to convert from Hex to RGB
R = int(HEX[1:3], 16)
G = int(HEX[3:5], 16)
B = int(HEX[5:7], 16)
For example, if your HEX color code is #4286f4, you can convert it to RGB like this:
R = int('FF', 16) = 255
G = int('00', 16) = 0
B = int('00', 16) = 0
So the RGB value for #FF0000
would be (255, 0, 0)
.
To convert from RGB to HSL, you can use the following formula:
R = rgb[0] / 255
G = rgb[1] / 255
B = rgb[2] / 255
Cmax = max(R, G, B)
Cmin = min(R, G, B)
L = (Cmax + Cmin) / 2
if Cmax == Cmin:
H = 0
S = 0
else:
if L < 0.5:
S = (Cmax - Cmin) / (Cmax + Cmin)
else:
S = (Cmax - Cmin) / (2 - Cmax - Cmin)
if Cmax == R:
H = (G - B) / (Cmax - Cmin)
elif Cmax == G:
H = 2 + (B - R) / (Cmax - Cmin)
else:
H = 4 + (R - G) / (Cmax - Cmin)
H = H * 60
if H < 0:
H += 360
So the HSL value for (255, 0, 0)
would be (0, 0, 0.5)
.
Converting RGB to HSL in Visual Studio Code
To convert an RGB color to HSL in Visual Studio Code, you can use the following formula:
hsl(hue, saturation, lightness)
Where hue
represents the color itself (e.g. red, green, blue, etc.), saturation
represents the intensity of the color, and lightness
represents the brightness of the color. These values can be calculated using the following algorithm:
- Normalize the RGB values by dividing them by 255.
- Calculate the maximum and minimum of the normalized RGB values.
- Calculate the lightness by adding the maximum and minimum and dividing by 2.
- If the maximum and minimum are equal, set the saturation and hue to 0.
- If the maximum and minimum are not equal, calculate the saturation and hue using the following formulas:
saturation = (max - min) / (1 - abs(max + min - 1))
hue = 60 * ((r - g) / (max - min)) + 120 (if max = b)
hue = 60 * ((g - b