Custom a slider is a common and versatile component used in web development to showcase multiple images or content in an interactive and visually appealing way. In this tutorial, we’ll guide you through the process of creating a functional slider using HTML for structure, CSS for styling, and JavaScript for the interactive behavior.
Table of Contents
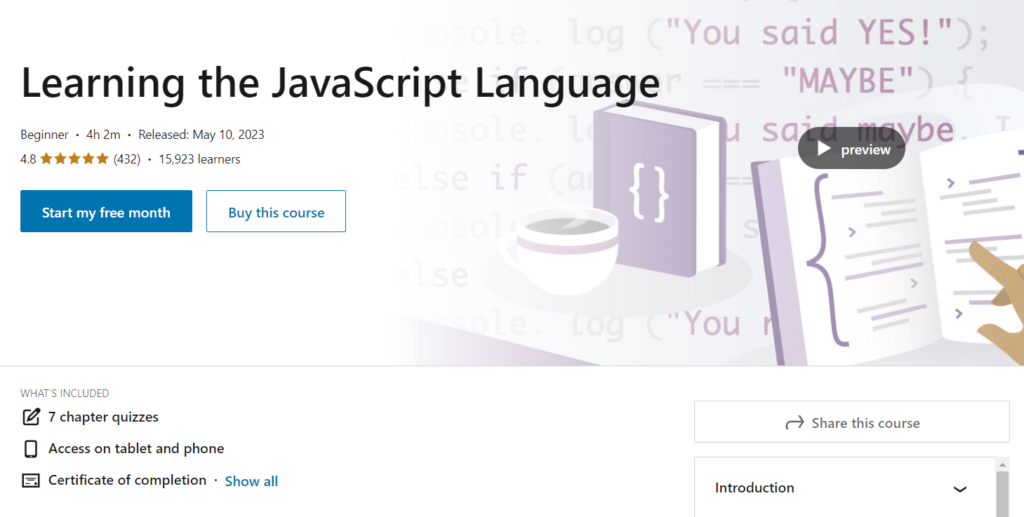
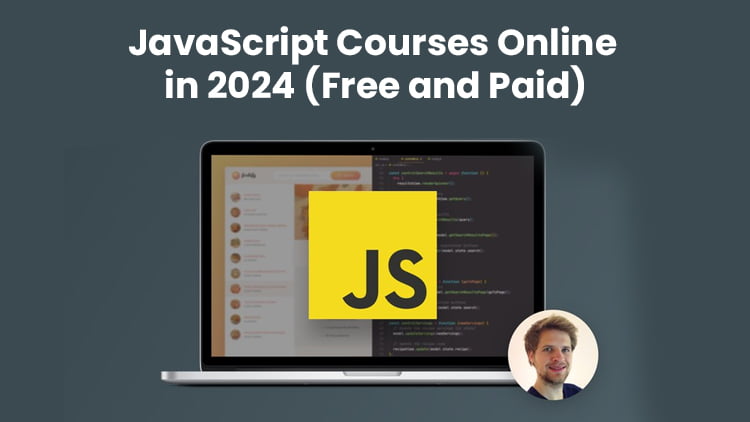
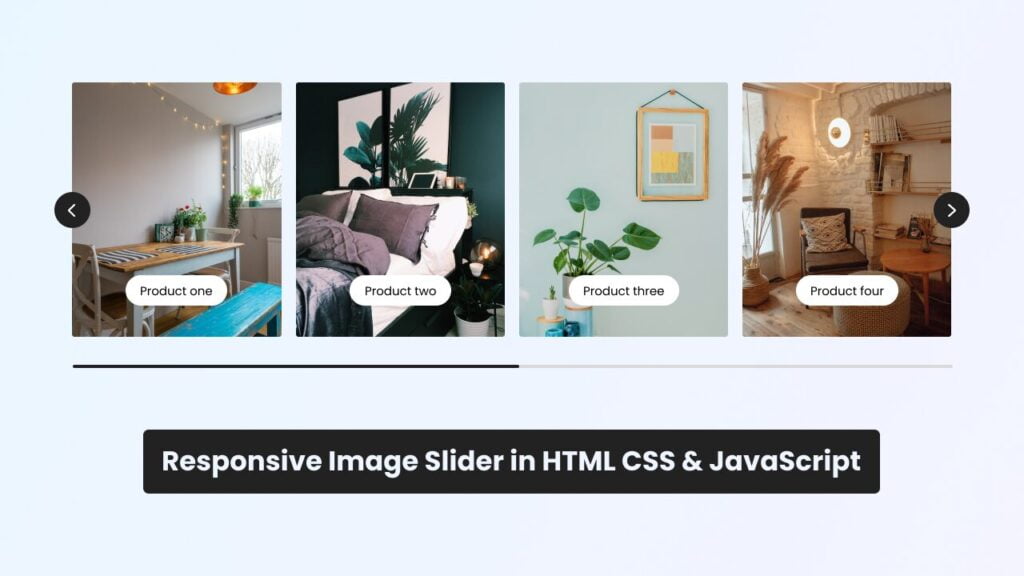
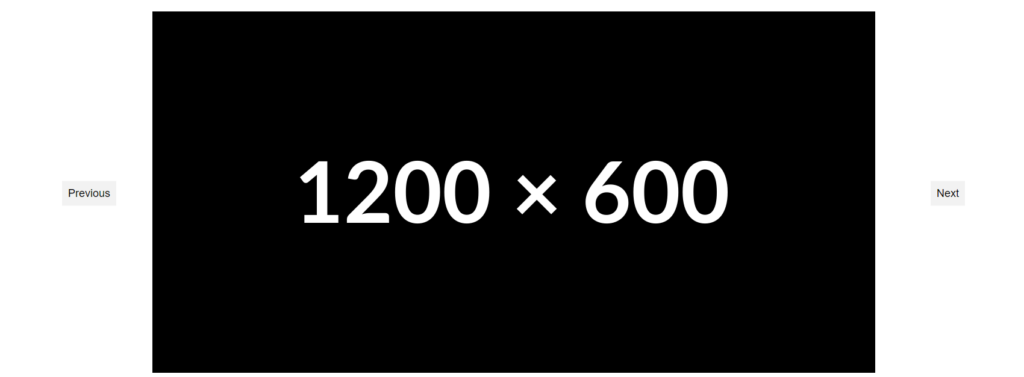
Introduction
Before we dive into the code, let’s briefly discuss the key components of custom a slider and what we aim to achieve in this tutorial.
A slider typically consists of a container that holds a series of slides, each containing different content (such as images or text). Users can navigate through these slides using navigation buttons, often labeled as “Previous” and “Next.”
For our tutorial, we’ll create a simple slider with three slides, navigation buttons, and a responsive layout.
Setting Up the HTML Structure for Custom a slider
First, let’s create the HTML structure for our Custom a slider. We’ll use a container div to hold the Custom a slider and two buttons for navigation. Open your HTML file and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Simple Slider</title>
</head>
<body>
<div class="slider-container">
<div class="slider">
<div class="slide" id="slide1"></div>
<div class="slide" id="slide2"></div>
<div class="slide" id="slide3"></div>
</div>
<button class="prev-btn" onclick="changeSlide(-1)">Previous</button>
<button class="next-btn" onclick="changeSlide(1)">Next</button>
</div>
<script src="script.js"></script>
</body>
</html>
Here, we have a container div with the class slider-container
, a div with the class slider
to hold our slides, and three slide divs with unique IDs (slide1
, slide2
, and slide3
). The “Previous” and “Next” buttons have corresponding classes (prev-btn
and next-btn
).
Applying CSS Styling
Now, let’s add some CSS to style our slider. Create a new file called styles.css
and include the following styles:
body {
font-family: 'Arial', sans-serif;
margin: 0;
padding: 0;
}
.slider-container {
position: relative;
width: 80%;
margin: auto;
overflow: hidden;
}
.slider {
display: flex;
transition: transform 0.5s ease-in-out;
}
.slide {
min-width: 100%;
height: 300px;
}
.prev-btn,
.next-btn {
position: absolute;
top: 50%;
transform: translateY(-50%);
font-size: 18px;
padding: 10px;
cursor: pointer;
}
.prev-btn {
left: 10px;
}
.next-btn {
right: 10px;
}
These CSS styles define the basic layout and appearance of our slider. The .slider-container
is set to 80% width, and the .slider
uses the flexbox model for horizontal scrolling. The .slide
class represents each individual slide, and the navigation buttons are styled to appear on the left and right sides of the container.
Implementing JavaScript Functionality
Now, let’s add the JavaScript code to handle the sliding functionality. Create a new file called script.js
and include the following code:
let currentSlide = 1;
function changeSlide(direction) {
const slider = document.querySelector('.slider');
const totalSlides = document.querySelectorAll('.slide').length;
if (direction === -1 && currentSlide > 1) {
currentSlide--;
} else if (direction === 1 && currentSlide < totalSlides) {
currentSlide++;
}
const translateValue = -100 * (currentSlide - 1) + '%';
slider.style.transform = 'translateX(' + translateValue + ')';
}
This JavaScript code introduces a variable currentSlide
to keep track of the currently visible slide. The changeSlide
function takes a parameter direction
(-1 for previous, 1 for next) and updates the currentSlide
accordingly. It then calculates the translation value based on the current slide, adjusting the slider’s position.
Testing and Further Customization
Now that we’ve set up the basic slider, open your HTML file in a web browser to see the result. You should be able to navigate through the slides using the “Previous” and “Next” buttons.
Feel free to customize the content of each slide, add more slides, or modify the styling to suit your project’s needs. You can also explore additional features such as automatic sliding, pagination, or navigation indicators.
other sliders – link
Conclusion
In this tutorial, we’ve walked through the process of creating a simple and functional slider using HTML, CSS, and JavaScript. Sliders are widely used in web development to showcase content in an engaging way, and understanding how to build one gives you the flexibility to enhance your projects with interactive elements.
As you continue to develop your skills, consider exploring more advanced slider implementations, such as integrating third-party libraries or incorporating CSS animations for smoother transitions. Remember to always test your sliders on different devices and browsers to ensure a consistent and responsive user experience. Happy coding!