Chrome extensions are a fantastic way to customize and enhance your browsing experience. With a basic understanding of HTML, CSS, and JavaScript, you can create your own Chrome extension from scratch. In this step-by-step guide, we’ll walk you through the process of creating a simple extension that changes the background color of any webpage.
Prerequisites:
Before you begin, ensure you have the following:
- A code editor (e.g., Visual Studio Code, Sublime Text).
- Google Chrome browser installed on your computer.
Step 1: Setting Up the Project for Chrome Extension
Create a new folder for your extension. Give it a name like “ColorChangerExtension.”
Step 2: Create the Manifest File
- Inside your project folder, create a file named
manifest.json
. - Open
manifest.json
in your code editor and add the following content:
{
"manifest_version": 2,
"name": "Color Changer Extension",
"version": "1.0",
"description": "Change the background color of any webpage.",
"permissions": ["activeTab"],
"browser_action": {
"default_popup": "popup.html",
"default_icon": {
"16": "icon16.png",
"48": "icon48.png",
"128": "icon128.png"
}
},
"icons": {
"16": "icon16.png",
"48": "icon48.png",
"128": "icon128.png"
},
"content_scripts": [
{
"matches": ["<all_urls>"],
"js": ["content.js"],
"run_at": "document_end"
}
]
}
Step 3: Designing the Popup for Chrome Extension
- Create a file named
popup.html
in the project folder. - Open
popup.html
and add the following content:
Color Changer Extension
Change Background Color
<!DOCTYPE html>
<html>
<head>
<title>Color Changer Extension</title>
<style>
body {
width: 150px;
text-align: center;
}
</style>
</head>
<body>
<h2>Change Background Color</h2>
<input type="color" id="colorPicker" value="#ffffff">
<script src="popup.js"></script>
</body>
</html>
Step 4: Adding JavaScript Logic
- Create a file named
popup.js
in the project folder. - Open
popup.js
and add the following JavaScript code:
document.addEventListener("DOMContentLoaded", function () {
const colorPicker = document.getElementById("colorPicker");
colorPicker.addEventListener("change", function () {
const color = colorPicker.value;
chrome.tabs.query({ active: true, currentWindow: true }, function (tabs) {
chrome.scripting.executeScript({
target: { tabId: tabs[0].id },
function: changeBackgroundColor,
args: [color],
});
});
});
function changeBackgroundColor(color) {
document.body.style.backgroundColor = color;
}
});
Step 5: Testing Your Extension
- Open Google Chrome and go to
chrome://extensions/
. - Enable “Developer mode” by toggling the switch in the top right corner.
- Click the “Load unpacked” button and select your extension folder.
- Your extension icon should now appear in the Chrome toolbar.
Step 6: Try It Out
- Visit any webpage and click on your extension icon.
- The popup will open, allowing you to pick a color. After selecting a color, the background color of the webpage should change accordingly.
More other html CSS blog here
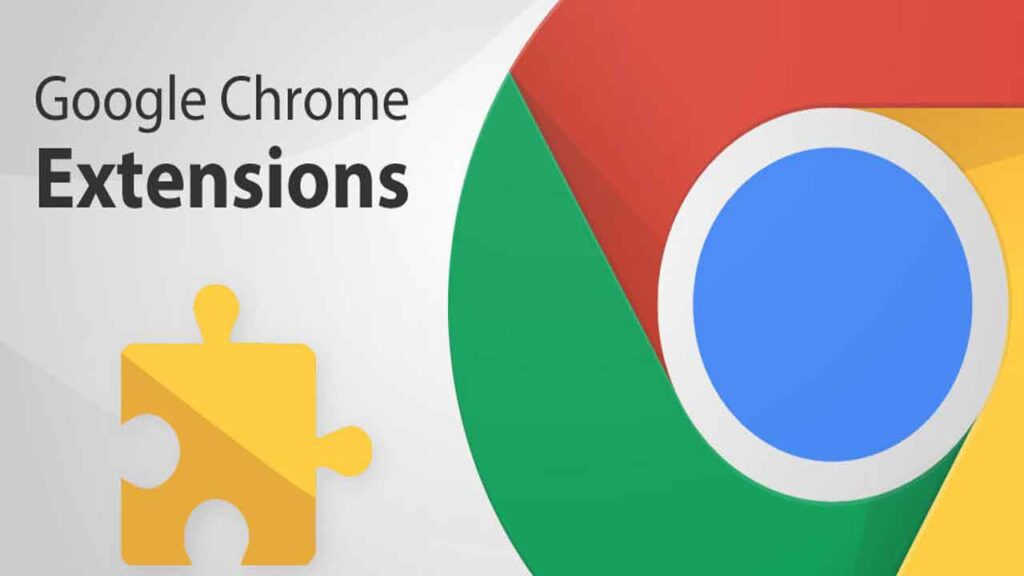
Conclusion
Congratulations! You’ve successfully created a simple Chrome extension using HTML, CSS, and JavaScript. This basic example demonstrates how you can leverage these technologies to build powerful browser enhancements. From here, you can explore more complex functionalities, integrate with APIs, and design extensions that cater to your specific needs. Enjoy experimenting and building unique extensions to make your browsing experience even more enjoyable and productive.