Form Validation are integral components of web development, enabling users to provide and submit their data. Nevertheless, assuring the precision and trustworthiness of this data remains paramount, highlighting the significance of form validation techniques.
Form validation encompasses the process of verifying that user-entered information within a form adheres to predetermined standards and is deemed legitimate. Its fundamental objective is to thwart the submission of deficient or unauthorized data.
This article aims to provide novice web developers with step-by-step instructions for implementing form validation utilizing HTML, CSS, and JavaScript. The journey commences with a foundational approach, instructing you in the crafting of a responsive form via HTML and CSS. Subsequently, we will delve into the realm of form validation, unraveling the adept utilization of JavaScript to accomplish this task with efficacy.
Form validation involves ensuring that the data entered by users in a form meets specific criteria and is valid. Its primary purpose is to prevent the submission of incomplete or invalid data.
The intention behind this blog article is to provide a comprehensive walkthrough for aspiring web developers, leading them through the intricacies of form validation employing HTML, CSS, and JavaScript. Beginning from the ground up, you’ll gain an understanding of fabricating a fluid and adaptable form utilizing HTML and CSS. Subsequently, we’ll plunge into the domain of form validation, delving into the adept deployment of JavaScript to implement it with utmost efficiency.
Furthermore, you’ll acquire the skill of toggling password visibility, revealing or concealing it upon clicking the toggle button. To witness the practical application of this form validation, proceed to the page’s lower section and engage the “View Live Demo” button for an interactive demonstration.
Guidelines to Establish Form Validation in HTML
To craft a form and execute validation using HTML, CSS, and pure JavaScript, adhere to the subsequent instructions meticulously:
- Create a folder. You can name this folder whatever you want, and inside this folder, create the mentioned files.
- Generate an index.html file. The file name must be index, and its extension should be .html.
- Formulate a thank-you.html file. The file name must be thank-you, and its extension should be .html.
- Craft a style.css file. The file name should be style, and its extension should be .css.
- Construct a script.js file. The file name should be script, and its extension should be .js.
Begin by incorporating the subsequent HTML codes into your index.html file to establish a fundamental form structure: This form encompasses fields for full name, email, password, date of birth, and gender. However, you have the flexibility to append or delete fields as per your preference.
<!DOCTYPE html>
<!-- Website - www.codingnepalweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Validation in HTML | CodingNepal</title>
<link rel="stylesheet" href="style.css">
<!-- Fontawesome CDN Link For Icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.1/css/all.min.css" />
</head>
<body>
<form action="thank-you.html">
<h2>Form Validation</h2>
<div class="form-group fullname">
<label for="fullname">Full Name</label>
<input type="text" id="fullname" placeholder="Enter your full name">
</div>
<div class="form-group email">
<label for="email">Email Address</label>
<input type="text" id="email" placeholder="Enter your email address">
</div>
<div class="form-group password">
<label for="password">Password</label>
<input type="password" id="password" placeholder="Enter your password">
<i id="pass-toggle-btn" class="fa-solid fa-eye"></i>
</div>
<div class="form-group date">
<label for="date">Birth Date</label>
<input type="date" id="date" placeholder="Select your date">
</div>
<div class="form-group gender">
<label for="gender">Gender</label>
<select id="gender">
<option value="" selected disabled>Select your gender</option>
<option value="Male">Male</option>
<option value="Female">Female</option>
<option value="Other">Other</option>
</select>
</div>
<div class="form-group submit-btn">
<input type="submit" value="Submit">
</div>
</form>
<script src="script.js"></script>
</body>
</html>
Next, add the following HTML codes to your thank-you.html
file: When the user fills out the form with valid data, they will be redirected to this HTML page. This page includes only a heading that says, “Thank you for filling out the form correctly.”.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Thanks page</title>
</head>
<body>
<h1>Thank you for filling out the form correctly.</h1>
</body>
</html>
Subsequently, incorporate the subsequent CSS snippets into your style.css file in order to design the form, enhancing its responsiveness and aesthetic appeal. Feel free to personalize these snippets according to your preferences by modifying attributes such as color, font, size, and other CSS properties.
@import url('https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;500;600;700&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Open Sans', sans-serif;
}
body {
display: flex;
align-items: center;
justify-content: center;
padding: 0 10px;
min-height: 100vh;
background: #1BB295;
}
form {
padding: 25px;
background: #fff;
max-width: 500px;
width: 100%;
border-radius: 7px;
box-shadow: 0 10px 15px rgba(0, 0, 0, 0.05);
}
form h2 {
font-size: 27px;
text-align: center;
margin: 0px 0 30px;
}
form .form-group {
margin-bottom: 15px;
position: relative;
}
form label {
display: block;
font-size: 15px;
margin-bottom: 7px;
}
form input,
form select {
height: 45px;
padding: 10px;
width: 100%;
font-size: 15px;
outline: none;
background: #fff;
border-radius: 3px;
border: 1px solid #bfbfbf;
}
form input:focus,
form select:focus {
border-color: #9a9a9a;
}
form input.error,
form select.error {
border-color: #f91919;
background: #f9f0f1;
}
form small {
font-size: 14px;
margin-top: 5px;
display: block;
color: #f91919;
}
form .password i {
position: absolute;
right: 0px;
height: 45px;
top: 28px;
font-size: 13px;
line-height: 45px;
width: 45px;
cursor: pointer;
color: #939393;
text-align: center;
}
.submit-btn {
margin-top: 30px;
}
.submit-btn input {
color: white;
border: none;
height: auto;
font-size: 16px;
padding: 13px 0;
border-radius: 5px;
cursor: pointer;
font-weight: 500;
text-align: center;
background: #1BB295;
transition: 0.2s ease;
}
.submit-btn input:hover {
background: #179b81;
}
In conclusion, append the provided JavaScript code to your script.js file. This code facilitates form validation, presents relevant error messages, and establishes the toggle mechanism for password visibility.
// Selecting form and input elements
const form = document.querySelector("form");
const passwordInput = document.getElementById("password");
const passToggleBtn = document.getElementById("pass-toggle-btn");
// Function to display error messages
const showError = (field, errorText) => {
field.classList.add("error");
const errorElement = document.createElement("small");
errorElement.classList.add("error-text");
errorElement.innerText = errorText;
field.closest(".form-group").appendChild(errorElement);
}
// Function to handle form submission
const handleFormData = (e) => {
e.preventDefault();
// Retrieving input elements
const fullnameInput = document.getElementById("fullname");
const emailInput = document.getElementById("email");
const dateInput = document.getElementById("date");
const genderInput = document.getElementById("gender");
// Getting trimmed values from input fields
const fullname = fullnameInput.value.trim();
const email = emailInput.value.trim();
const password = passwordInput.value.trim();
const date = dateInput.value;
const gender = genderInput.value;
// Regular expression pattern for email validation
const emailPattern = /^[^ ]+@[^ ]+\.[a-z]{2,3}$/;
// Clearing previous error messages
document.querySelectorAll(".form-group .error").forEach(field => field.classList.remove("error"));
document.querySelectorAll(".error-text").forEach(errorText => errorText.remove());
// Performing validation checks
if (fullname === "") {
showError(fullnameInput, "Enter your full name");
}
if (!emailPattern.test(email)) {
showError(emailInput, "Enter a valid email address");
}
if (password === "") {
showError(passwordInput, "Enter your password");
}
if (date === "") {
showError(dateInput, "Select your date of birth");
}
if (gender === "") {
showError(genderInput, "Select your gender");
}
// Checking for any remaining errors before form submission
const errorInputs = document.querySelectorAll(".form-group .error");
if (errorInputs.length > 0) return;
// Submitting the form
form.submit();
}
// Toggling password visibility
passToggleBtn.addEventListener('click', () => {
passToggleBtn.className = passwordInput.type === "password" ? "fa-solid fa-eye-slash" : "fa-solid fa-eye";
passwordInput.type = passwordInput.type === "password" ? "text" : "password";
});
// Handling form submission event
form.addEventListener("submit", handleFormData);
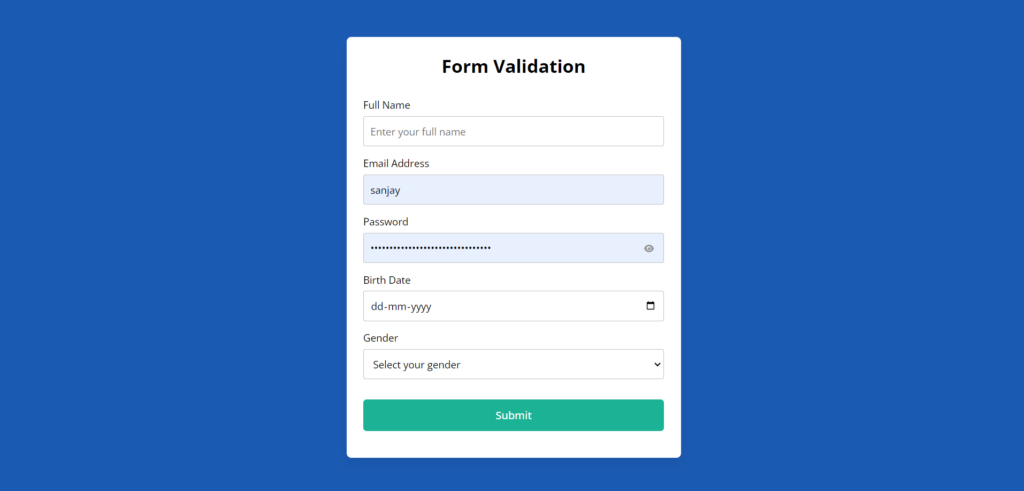
Conclusion and Final Words
To conclude, we initiated the construction of the form using HTML, outlining the essential input fields along with their attributes. Subsequently, we utilized CSS to imbue style upon elements like form fields, labels, and buttons. Lastly, JavaScript was employed to actualize form validation logic, ensuring the entry of valid user data.
By adhering to the guidance furnished within this blog post, you should now possess a fully operational form replete with validation functionalities. To further enrich your proficiencies in web development, I urge you to delve into and experiment with the diverse array of form types and validations accessible on this website.
Should you encounter any challenges during the form creation process or if your code fails to perform as anticipated, you have the option to acquire the source code files for this form free of charge by selecting the Download button. Furthermore, a live demonstration is available for your perusal via the View Live button.
Van Mosley