in this article you can know JavaScript Concepts, Do you ever wonder how much of JavaScript you truly comprehend? You might be familiar with writing functions, grasping basic algorithms, and even creating classes. But have you ever encountered the concept of a typed array?
Don’t fret if you haven’t explored all these ideas yet, as they may become crucial in your future career endeavors. That’s why I suggest saving this list for reference, as it’s likely that you’ll come across one of these topics at some point, and having a tutorial on hand will be immensely beneficial in gaining a complete understanding.
Keep in mind that this list draws inspiration from a specific repository, which served as a valuable source for its compilation.
1. Closures – JavaScript Concepts
In JavaScript, variables declared using var
, let
, or const
have different levels of scope:
- Global Scope: Variables declared outside any function or block are considered to be in the global scope. They are accessible from any part of the code.
- Function Scope: Variables declared within a function using
var
are scoped to that function. They are not accessible outside the function. - Block Scope: Variables declared with
let
orconst
within a block (inside curly braces{}
) are scoped to that block. They are not accessible outside the block.
Now, let’s dive into JavaScript Concepts closures:
A Closure is a function that retains access to variables from its containing (enclosing) function’s scope, even after the enclosing function has finished executing.
In simpler terms, a closure allows a function to “remember” the environment in which it was created, including all the variables that were in scope at the time of its creation.
Here’s a basic example to illustrate closures:
function outerFunction() {
let outerVariable = 'I am from the outer function';
function innerFunction() {
console.log(outerVariable); // The inner function has access to outerVariable
}
return innerFunction;
}
const closureFunction = outerFunction(); // closureFunction now holds the innerFunction
closureFunction(); // This will log: "I am from the outer function"
In this example, createCounter
returns an object with three methods, and each method forms a closure with the private count
variable, allowing us to create a counter with private state.
Closures are a powerful tool in JavaScript and are commonly used in many scenarios to manage state, encapsulate behavior, and create modular and reusable code.
2.High Order Functions – JavaScript Concepts
f course! In JavaScript, high-order functions are functions that can take other functions as arguments or return functions as their results. In other words, they treat functions as first-class citizens. This concept is a fundamental part of functional programming, which allows you to write more concise and flexible code.
To better understand high-order functions, let’s break down the two main aspects: functions that take other functions as arguments and functions that return functions.
- Functions that take other functions as arguments: In JavaScript, you can pass a function as an argument to another function, just like you would pass any other data type (e.g., strings, numbers). Here’s a simple example:
// A high-order function that takes another function as an argument
function operateOnNumber(number, operation) {
return operation(number);
}
function double(x) {
return x * 2;
}
function square(x) {
return x * x;
}
const num = 5;
console.log(operateOnNumber(num, double)); // Output: 10
console.log(operateOnNumber(num, square)); // Output: 25
In the example above, operateOnNumber
is a high-order function that takes two arguments: a number and a function (operation
). When you call operateOnNumber
and pass double
or square
as the operation
, it applies that function to the number and returns the result.
- Functions that return functions: In JavaScript, functions can also return other functions. This allows you to create more specialized functions or function factories. Here’s an example:
// A high-order function that returns a function
function createMultiplier(multiplier) {
return function (number) {
return number * multiplier;
};
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
In this example, createMultiplier
is a high-order function that takes a multiplier
as an argument and returns a new function. The returned function takes a number
argument and multiplies it by the provided multiplier
.
in this JavaScript Concepts High-order functions are widely used in JavaScript and are essential for functional programming paradigms. They enable you to write more modular and reusable code by abstracting common functionality into higher-order functions. Examples of built-in high-order functions in JavaScript include map
, filter
, reduce
, forEach
, and sort
.
3.Promises – JavaScript Concepts
Promises in JavaScript Concepts are a way to handle asynchronous operations, such as fetching data from a server, reading files, or making API calls. Prior to the introduction of Promises, managing asynchronous code was done using callbacks, which could often lead to complex and hard-to-maintain code structures. Promises were introduced to provide a more organized and manageable way of handling asynchronous tasks.
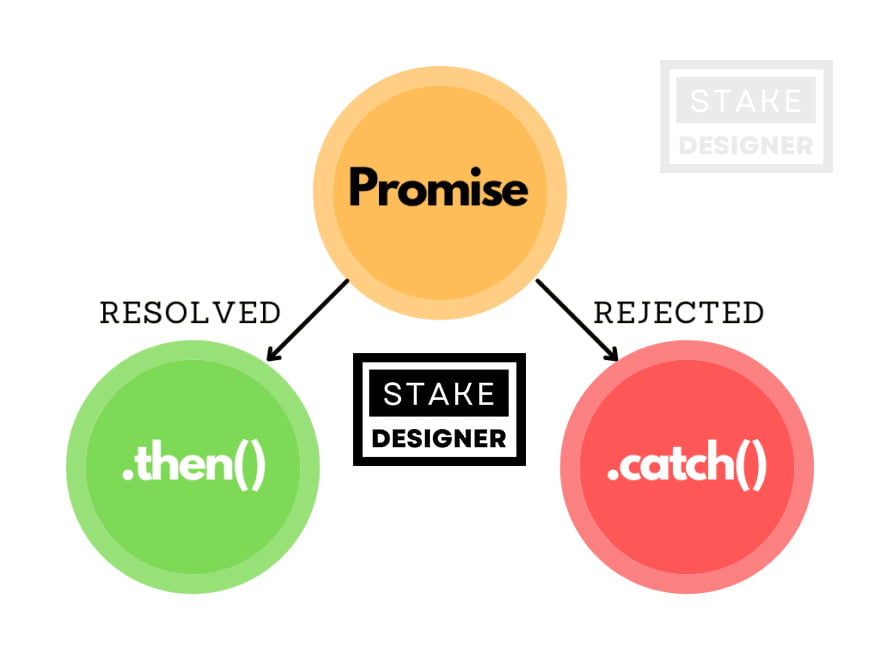
A Promise is an object that represents a value that may not be available yet but will be resolved or rejected at some point in the future. It can be in one of three states:
- Pending: The initial state when the Promise is created. The Promise is neither resolved nor rejected at this point.
- Fulfilled (Resolved): The state when the asynchronous operation is successfully completed. The Promise returns a value, and the associated success callback, known as
resolve
, is called. - Rejected: The state when the asynchronous operation encounters an error. The Promise returns a reason for failure, and the associated error callback, known as
reject
, is called.
The syntax to create a Promise in JavaScript Concepts looks like this:
const myPromise = new Promise((resolve, reject) => {
// Asynchronous code here
// If the operation is successful, call 'resolve' with the result
// If there's an error, call 'reject' with the reason for failure
});
Let’s see an example of a Promise in action:
const fetchData = () => { return new Promise((resolve, reject) => { // Simulate an API call with a timeout setTimeout(() => { const data = { name: "John", age: 30 }; // Assuming the data was fetched successfully resolve(data); // If an error occurs during the fetch, use 'reject' instead }, 2000); }); }; fetchData() .then((data) => { console.log("Data fetched successfully:", data); }) .catch((error) => { console.error("Error fetching data:", error); });
In the example above, fetchData
is a function that returns a Promise. When fetchData()
is called, the asynchronous operation (simulated with a setTimeout
) starts. After two seconds, the Promise will be resolved with the data
object, and the then
block will be executed. If there’s an error during the asynchronous operation, the Promise will be rejected, and the catch
block will be executed.
Promises help to make the code more readable and maintainable, especially when dealing with multiple asynchronous tasks. They also enable better error handling by centralizing error management in a single catch
block at the end of the Promise chain. Additionally, Promises can be chained together to perform sequential or parallel asynchronous operations.
5.Recursion – JavaScript Concepts
Consider this post as a series of learning exercises. These examples are designed to make you think — and, if I’m doing it right, maybe expand your understanding of functional programming a little bit.
Tutorials
- 📜 Recursion in JavaScript — Kevin Ennis
- 📜 Understanding Recursion in JavaScript — Zak Frisch
- 📜 Learn and Understand Recursion in JavaScript — Brandon Morelli
4 Clean Code – JavaScript Concepts
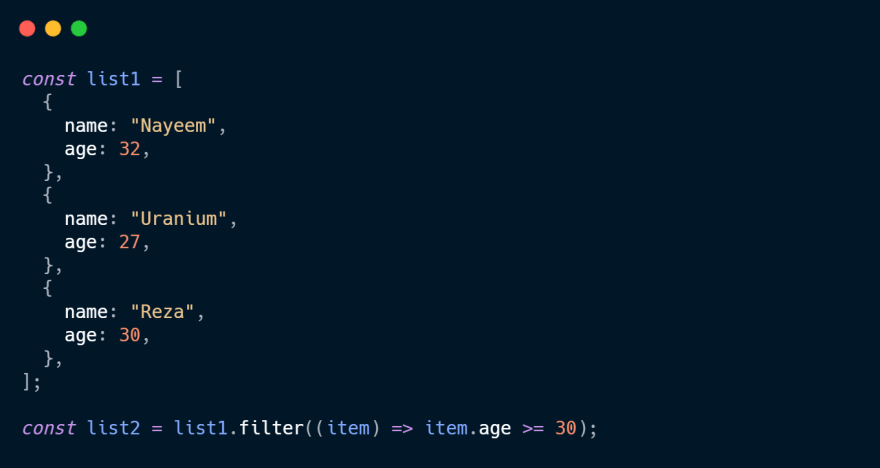
Writing clean, understandable, and maintainable code is a skill that is crucial for every developer to master.
More JavaScript project JavaScript Project